일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- 나만무
- TiL
- 크래프톤 정글
- 모션비트
- pintos
- corou
- 자바
- Vue.js
- JavaScript
- 백준
- defee
- Flutter
- 자바스크립트
- 소켓
- userprog
- 프로그래머스
- 스택
- Java
- CSS
- 리액트
- 핀토스
- HTML
- 알고리즘
- 사이드프로젝트
- 크래프톤정글
- 큐
- 정보처리기사
- 4기
- 코드트리
- 시스템콜
- Today
- Total
문미새 개발일지
24.08.26 day53 작업 일지 본문
본격적인 기능은 나중이지만 마이페이지와 장바구니 UI도 틀은 완성했다. 마이페이지 경우에는 기능 구현을 어디까지 할지는 모르겠지만 페이지의 구색은 갖춰야 하니 기획한 모든 메뉴는 만들어놨다. 여기서 추가할 게 있으면 추가하고 필요없는 부분은 추후에 수정할 계획이다.
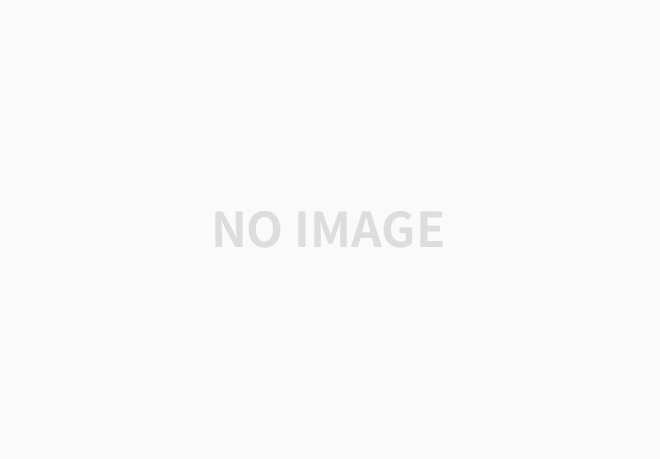
상단 3개 기능은 구현가능하지만 하단 4개 기능은 제대로된 서비스가 될 경우에 사용가능한 거라 이 부분은 페이지의 구색으로만 만들어놨다.
import styled from "styled-components";
import AboutHeader from "../components/common/aboutHeader";
import Profile from "../components/mypage/profile";
import Option from "../components/mypage/option";
const Mypage: React.FC = () => {
return (
<>
<MypageWrapper>
<AboutHeader Title={"마이페이지"} />
<Profile />
<Option />
</MypageWrapper>
</>
);
};
export default Mypage;
const MypageWrapper = styled.div`
width: 100%;
`;
마이페이지는 공용컴포넌트인 헤더와 프로필 표시 부분 그리고 옵션 부분으로 이루어져있다.
import "../../scss/mypage/profile.scss";
const Profile: React.FC = () => {
return (
<>
<div className="profileWrapper">
<div className="profileImg">
<div>
<img src="#" alt="" />
</div>
<p>재남</p>
</div>
<div className="selectFilter">
<div>건성</div>
<div>남성</div>
<div>30대</div>
<div>민감성</div>
<div>겨울쿨</div>
<div>등</div>
<div>등등</div>
</div>
<button>프로필 수정</button>
</div>
</>
);
};
export default Profile;
이번 프로젝트는 atomic design처럼 하나씩 쪼개지 않고 파트별로 나누기로 했다. 해당 컴포넌트는 scss 방식으로 작성해서 css를 따로 작성했다.
프로필의 태그 부분은 지그재그로 구현할려 했었으나 개수가 같아질 경우 같은 위치로 들어가야 하기 때문에 어색한 부분이 있어서 grid로 묶어놨다.
import styled from "styled-components";
import SettingBox from "./settingBox";
const Option: React.FC = () => {
return (
<>
<OptionWrapper>
<SettingWrapper1>
<SettingBox name="주문 내역" />
<SettingBox name="취소/반품/교환 내역" />
<SettingBox name="나의 맞춤 정보" />
</SettingWrapper1>
<SettingWrapper2>
<SettingBox name="고객센터" />
<SettingBox name="1:1 문의 내역" />
<SettingBox name="상품 문의 내역" />
<SettingBox name="공지사항" />
</SettingWrapper2>
<Logout>로그아웃</Logout>
</OptionWrapper>
</>
);
};
export default Option;
const OptionWrapper = styled.div`
width: 90%;
margin: 30px auto;
`;
const SettingWrapper1 = styled.div`
width: 100%;
margin-bottom: 70px;
`;
const SettingWrapper2 = styled.div`
width: 100%;
border-bottom: 5px solid #e8e8e8;
margin-bottom: 10px;
`;
const Logout = styled.span`
font-size: 12px;
color: #848484;
text-decoration: underline;
cursor: pointer;
`;
옵션 부분은 아직 해당 기능을 구현하지 않아서 div 구조만 만들어놨고 이 후 기능 구현할 때 navigate로 이동시킬 계획이다.
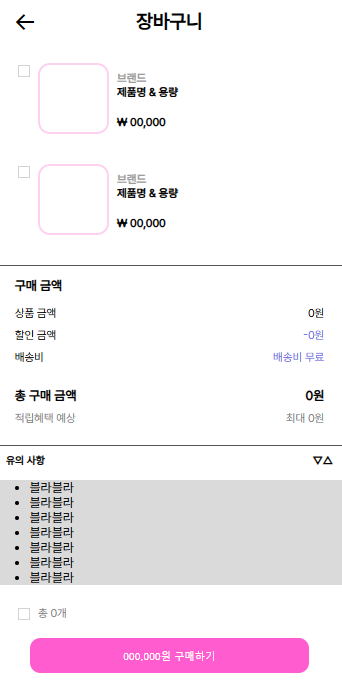
장바구니 페이지는 헤더, 장바구니 목록, 금액 정리, 유의 사항, 구매 버튼으로 되어있다.
헤더는 기존과 동일하고 장바구니 목록은 하나의 물품 정보 구조를 만들었다.
이 후 데이터를 가져올 때 데이터 개수를 map으로 받아와서 뿌릴 예정
import "../../scss/mart/martList.scss";
const MartList: React.FC = () => {
return (
<>
<div className="martListWrapper">
<div className="martItem">
<label>
<input type="checkbox" name="" id="" />
<div className="martItemWrapper">
<div className="martItemImg">
<img src="#" alt="" />
</div>
<div className="martItemInfo">
<span>브랜드</span>
<span>제품명 & 용량</span>
<p>₩ 00,000</p>
</div>
</div>
</label>
</div>
<div className="martItem">
<label>
<input type="checkbox" name="" id="" />
<div className="martItemWrapper">
<div className="martItemImg">
<img src="#" alt="" />
</div>
<div className="martItemInfo">
<span>브랜드</span>
<span>제품명 & 용량</span>
<p>₩ 00,000</p>
</div>
</div>
</label>
</div>
</div>
</>
);
};
export default MartList;
금액 정리 부분은 상품 금액 외에는 아직 정리해둔 사안이 없어서 사용 부분은 상품 금액과 총 구매 금액 부분 뿐일 것 같다. 나머지는 그냥 다른 페이지처럼 보이게 간지용으로 작성
import "../../scss/mart/priceList.scss";
const PriceList: React.FC = () => {
return (
<>
<div className="priceListWrapper">
<div className="priceListBox">
<h3>구매 금액</h3>
<div>
<span>상품 금액</span>
<span>0원</span>
</div>
<div>
<span>할인 금액</span>
<span>-0원</span>
</div>
<div>
<span>배송비</span>
<span>배송비 무료</span>
</div>
<div>
<span>총 구매 금액</span>
<span>0원</span>
</div>
<div>
<span>적립혜택 예상</span>
<span>최대 0원</span>
</div>
</div>
</div>
</>
);
};
export default PriceList;
하단의 유의 사항은 상업 사이트가 명시해야 하는 규칙인데 이건 다른 페이지에서 긁어 올 예정이여서 냅둬놨다.
import styled from "styled-components";
const BuyBtn: React.FC = () => {
return (
<>
<BuyBtnWrapper>
<BuyCheck>
<label>
<input type="checkbox" />
<span>총 0개</span>
</label>
</BuyCheck>
<button>000,000원 구매하기</button>
</BuyBtnWrapper>
</>
);
};
export default BuyBtn;
const BuyBtnWrapper = styled.div`
width: 90%;
margin: 0 auto;
display: flex;
flex-direction: column;
align-items: center;
button {
width: 90%;
margin: 20px 0;
background-color: #ff5cd0;
border: none;
padding: 13px 0;
border-radius: 13px;
font-size: 13px;
color: white;
}
`;
const BuyCheck = styled.div`
width: 100%;
label {
display: flex;
input[type="checkbox"] {
appearance: none; /* 기본 체크박스 스타일 제거 */
width: 15px;
height: 15px;
border: 1px solid lightgray;
cursor: pointer;
margin-right: 10px;
&:checked {
background-color: rgba(255, 164, 228, 0.5);
border-color: rgba(255, 164, 228, 0.5);
}
&:checked::after {
content: "✓";
display: block;
color: black;
text-align: center;
line-height: 13px;
font-size: 15px;
}
}
span {
font-size: 14px;
line-height: 1.5;
color: #848484;
}
}
`;
구매 버튼은 총 물품 개수와 구매하기 버튼으로 이루어져있는데, 총 물품 개수가 input 체크박스 형식으로 되어있어 일단 체크박스로 만들어놨다. 근데 체크박스가 무슨 기능을 할지는 아직 몰라서 백엔드 팀원과 회의를 통해 조율해야 한다.
버튼은 총 금액 만큼 결제 페이지로 이동시키는 역할을 하는데 이 부분은 결제 api가 구현되야 손을 더 댈 수 있다.
grid가 생각보다 매우 유용하다 지금까지 flex만 주구장창 써왔는데 수평의 규칙적인 div구조가 있는 부분은 grid가 flex보다 쓰기 쉬운 것 같다.
'개발 TIL' 카테고리의 다른 글
24.08.28 day55 작업 일지 (2) | 2024.08.28 |
---|---|
24.08.27 day54 작업 일지 (0) | 2024.08.27 |
24.08.23 day52 캡슐화, 미들웨어 (0) | 2024.08.23 |
24.08.22 day51 작업 일지 (0) | 2024.08.22 |
24.08.21 day50 공용 컴포넌트 작성 방식 (0) | 2024.08.21 |